Table of contents
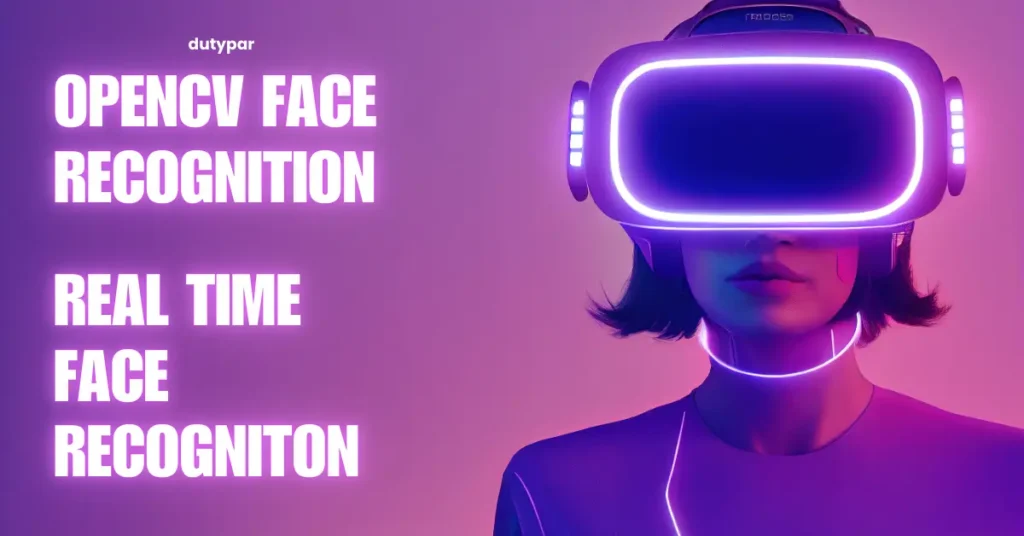
Face recognition technology has been advancing rapidly in recent years, becoming an essential tool in various fields such as security, healthcare, marketing, and even entertainment. Among the many libraries and frameworks available for face recognition, OpenCV stands out as one of the most popular and versatile. In this article, we will delve into OpenCV face recognition, how it works, and how you can implement it in your own projects.
What is OpenCV?
OpenCV (Open Source Computer Vision Library) is an open-source computer vision and machine learning software library. Originally developed by Intel, OpenCV offers a comprehensive suite of tools for real-time image processing and computer vision tasks. With its easy-to-use interface and extensive functionalities, OpenCV has become a go-to solution for developers, researchers, and engineers working on computer vision projects. Among its many capabilities, OpenCV face recognition is one of the most sought-after features due to its simplicity and effectiveness.
How Does OpenCV Face Recognition Work?
OpenCV face recognition leverages computer vision algorithms to detect and recognize human faces in digital images and videos. It uses machine learning techniques, including deep learning, to analyze facial features and match them against a database of known faces. The process of OpenCV face recognition can be broken down into several key steps:
- Face Detection: The first step in OpenCV face recognition is to detect faces in an image or video. OpenCV provides several pre-trained models and classifiers, such as Haar cascades and the deep learning-based DNN module, that can quickly identify faces. The Haar cascade classifier, for example, uses a series of rectangular filters to detect facial features like the eyes, nose, and mouth.
- Feature Extraction: Once the face is detected, the next step involves extracting key facial features. These features are unique points on the face that remain relatively unchanged, such as the distance between the eyes, the width of the nose, and the shape of the mouth. OpenCV uses algorithms like Local Binary Patterns Histogram (LBPH), Eigenfaces, and Fisherfaces to extract these features.
- Face Recognition: In the recognition phase, OpenCV compares the extracted facial features against a pre-existing database of known faces. If a match is found within a certain threshold, the system identifies the person. OpenCV supports multiple face recognition algorithms, each suited for different scenarios. For instance, LBPH is robust against variations in lighting and facial expressions, making it ideal for real-world applications.
- Post-Processing and Output: After successful recognition, the system can perform various actions based on the requirements, such as granting access to a secure area, marking attendance, or triggering an alert. OpenCV provides tools to annotate the recognized faces with names or IDs, making it easier to interpret the results.
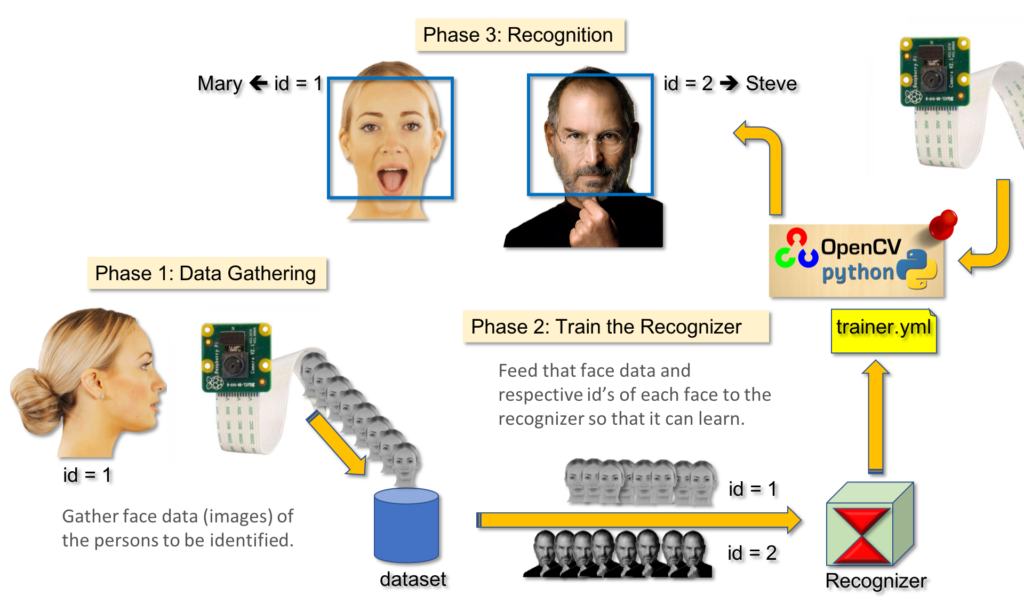
Implementing OpenCV Face Recognition: A Step-by-Step Guide
Implementing OpenCV face recognition in your own project is relatively straightforward, thanks to the library’s extensive documentation and user-friendly APIs. Here is a step-by-step guide to get you started with OpenCV face recognition:
- Install OpenCV: Before you can start with face recognition using openCV, you need to install the OpenCV library. You can do this using Python’s package manager, pip, by running the command:
pip install opencv-python
- Import Required Libraries: Once OpenCV is installed, you need to import the necessary libraries in your Python script. At a minimum, you will need to import
cv2
for OpenCV andnumpy
for handling arrays:
import cv2
import numpy as np
- Load the Pre-trained Model: OpenCV comes with several pre-trained models for face detection. For this guide, we will use the Haar cascade classifier. You can download the
haarcascade_frontalface_default.xml
file from the OpenCV GitHub repository and load it into your script:
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
- Capture or Load an Image/Video: To perform OpenCV face recognition, you need an input image or video. You can either load an existing image or capture a real-time video feed using your device’s camera:
# Load an image
img = cv2.imread('sample_image.jpg')
# Capture video feed
cap = cv2.VideoCapture(0)
- Detect Faces in the Image/Video: Use the loaded classifier to detect faces in the image or video frame. The
detectMultiScale
method scans the input for faces and returns the coordinates of detected faces:
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Convert to grayscale
faces = face_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5)
# Draw rectangles around detected faces
for (x, y, w, h) in faces:
cv2.rectangle(img, (x, y), (x+w, y+h), (255, 0, 0), 2)
- Recognize Faces Using LBPH: For actual OpenCV face recognition, you need to train a model on a dataset of known faces. The LBPH algorithm is widely used for this purpose due to its efficiency and accuracy. You can use the
cv2.face.LBPHFaceRecognizer_create()
method to create and train the recognizer:
recognizer = cv2.face.LBPHFaceRecognizer_create()
recognizer.train(training_images, labels) # training_images and labels should be pre-defined
- Display the Results: Finally, display the image or video frame with the recognized faces:
cv2.imshow('Face Recognition', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
Applications of OpenCV Face Recognition
OpenCV face recognition finds applications in a wide range of industries and use cases:
- Security and Surveillance: Organizations use OpenCV face recognition to enhance security by automatically identifying unauthorized personnel and granting access only to authorized individuals.
- Attendance Systems: Schools, universities, and companies implement face recognition using openCVfor automated attendance systems, reducing the need for manual record-keeping.
- Marketing and Retail: Retailers use face recognition to analyze customer demographics and shopping patterns, allowing them to offer personalized shopping experiences.
- Healthcare: face recognition using openCV helps in patient identification, ensuring that the right treatments and medications are administered.
- Smart Devices: Face recognition is widely used in smartphones and other smart devices for user authentication and personalized experiences.
Advantages and Limitations of OpenCV Face Recognition
Like any technology, OpenCV face recognition comes with its own set of advantages and limitations:
Advantages:
- Open Source: Being open source, OpenCV face recognition is accessible to everyone, allowing for easy experimentation and customization.
- Wide Range of Algorithms: OpenCV supports multiple face recognition algorithms, providing flexibility to choose the best one for a given application.
- Ease of Integration: OpenCV can easily integrate with other libraries, frameworks, and languages, making it versatile for various development environments.
Limitations:
- Dependence on Lighting Conditions: OpenCV face recognition can struggle in poor lighting or with low-quality images, affecting accuracy.
- Privacy Concerns: The use of face recognition technology raises privacy concerns, and developers must be mindful of ethical and legal implications.
- Computationally Intensive: Real-time face recognition can be computationally intensive, requiring powerful hardware for efficient performance.
Conclusion
OpenCV face recognition is a powerful tool for developers and researchers looking to implement face recognition capabilities in their projects. With its robust set of features, open-source nature, and flexibility, OpenCV makes it easier to develop applications that can detect, recognize, and analyze faces. However, it is essential to consider its limitations and ethical concerns while deploying face recognition using openCV in real-world applications.
Whether you are a beginner or an experienced developer, exploring OpenCV face recognition will undoubtedly enhance your skill set and open new possibilities in the world of computer vision. So, why not get started today and see what you can create with face recognition using openCV ?
This article takes the Coding of OpenCV as a reference from this website: